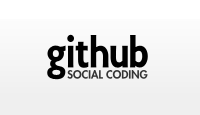
Yes, finally I’ve joined the masses on Github and I plan to sporadically share special things on there, starting with the following recent developments:
“literalHTML”
A tiny but powerful modification allowing you to specify HTML/DOM structures inline in your JavaScript code, an example:
var className = 'active'; var myMenu = | <ul> <li class="{className}">Item 1</li> <li>Item 2</li> <li>Item 3</li> </ul> |; // myMenu is now a DOM object: myMenu.nodeName; // "ul" myMenu.childNodes; // [ <li.active>, <li>, <li> ] // Do something: jQuery('body').append(myMenu); |
“getDescendants”
Something I required for a project; a function to gather all descendant-elements down until a certain depth is reached:
<div> <ul> <li>Something <strong>interesting</strong></li> </ul> <p>Something <span>else</span></p> </div> |
Examples:
var div = document.getElementsByTagName('div')[0]; getDescendants(div, 1); // -> [ <ul>, <p> ] // i.e. children getDescendants(div, 2); // -> [ <ul>, <p>, <li>, <span> ] // i.e. children + grandchildren getDescendants(div, 3); // -> [ <ul>, <p>, <li>, <span>, <strong> ] // i.e. children + grandchildren + great-grandchildren |
I’ll hopefully be adding to the repositroy with new exciting discoveries in the near future; visit my profile and “follow me” to stay updated!
Thanks for reading! Please share your thoughts with me on Twitter. Have a great day!
I made the move from SVV to git not that long ago, never looked back! oh and github is awsome, i’m even hosting the code for my blog there as a public repo 🙂
I like literalHTML.. it could be the starting point to implement E4X crossbrowser.. why not?
http://en.wikipedia.org/wiki/ECMAScript_for_XML
I noticed in the literalHTML code you’re using $.globalEval(). I can’t seem to find any documentation about it. What’s the advantage of using it instead of eval()?
@Daniel, agreed; I’m really starting to like Github, and Git generally!
@Valentino, E4X was actually the inspiration for literalHTML…
@Corey, I considered using
eval
but I thought it better to execute the script in a global context; after all, that’s how the:literalHTML
scripts are defined. Also$.globalEval
uses a much more harmless method of execution – it simply injects a new script element containing the code to be evaluated. To be honest I would feel perfectly comfortable usingeval
or(new Function())()
but I thought I should take advantage of what jQuery had to offer.[…] succuessfully implementing and using literalHTML (discussed here) I decided to have a go at creating a reusable basis for adding new enhancements just like it; your […]