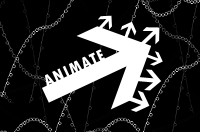
jQuery’s animation capabilities are normally either overlooked or overused. Some people consider animation on the web to be a fruitless endeavor while others seem to never get enough of it. Yet most don’t realise what jQuery allows you to do; it’s no longer just about accordions and slideshows!
The animate
method is incredibly versatile; it doesn’t have to manipulate a CSS property; any property of any object can be manipulated. This allows us to do things like this:
var from = {property: 0}; var to = {property: 100}; jQuery(from).animate(to, { duration: 100, step: function() { console.log( 'Currently @ ' + this.property ); } }); |
The step
function you see is an optional property of the also-optional object argument (the second one we’re passing to animate()
). If the step
function exists jQuery will run it on every single step of any animaton. This gives you limitless control, allowing you to manipulate any number of things.
The News Ticker
I know it’s an old and boring effect but I can’t resist; jQuery makes it so damn easy!
var text = 'jQuery is simply awesome! Blah blah blah!'; var span = $('<span/>').appendTo('body'); jQuery({count:0}).animate({count:text.length}, { duration: 1000, step: function() { span.text( text.substring(0, Math.round(this.count)) ); } }); |
Did you notice the easing!?
Easing
Another commonly overlooked feature of jQuery animation is easing. There are two places you can specify what easing to utilise; either as the third argument or as a property to the alternative two-argument call (see the docs):
var duration = 1000; var easing = 'linear'; var callback = function(){}; /* #1 */ jQuery('something').animate({prop:1}, duration, easing, callback); /* #2 */ jQuery('something').animate({prop:1},{ easing: easing, duration: duration, complete: callback }); |
Only two easing “equations” are bundled with jQuery, “linear” and “swing”. By default jQuery uses “swing”. There are many additional “equations” available. You just need to include jquery.easing.js
and you can use them in all your animations!
When I originally stumbled upon the easing plugin I really only wanted one thing; I clear way to illustrate what each easing-method did! I recently setup a page to do just that! Check it out!
Ok, this post wasn’t very long (the easing demo took longer than I anticipated); the gist of it is: There are still many undiscovered secrets within jQuery… “animate()” being one of them.
Thanks for reading! Please share your thoughts with me on Twitter. Have a great day!
I did not know about the step argument, thank you for sharing!
Your easing demo isn’t quite finished…
Jquery fails to load..
Having said that, I thought animate() only worked on css. The fact that it works on other properties is a very interesting idea. I may need to mess with this sometime.
The easing demo doesn’t work because it’s trying to load jquery from http://localhost/jquery
Ah, sorry about that, fixed now! 🙂
That demo is pretty neat, took me a short while to figure out what was going on exactly. It will be useful on the next project that I use spiffy JQuery UI on.
I created my own little take on JQuery animate() function. Pointless but fun 🙂 http://playground.adrianwestlake.com/jquery/hello/
Nice small Tutorial James! If you are interested in more jQuery, you don’t have to miss the following two articles: “Creative Image-Galleries by jQuery” or “jQuery – The easy way to navigate”
Really cool, i am using the Flock browser and linear is by far the smoothest animation and easiest on the eyes.
I should take into consideration that the box is “easing down” with an image in it, and wouldn’t be doing so in every situation. Thanks for this.
[…نوشتهای از James Padolsey برای پویانمایی خاصیتهای اشیا…]
Thank you for sharing this information
……
Thanks for the tips. I love jQuery and its always good to learn something new.
Cheers
Hey James! Nice article here. Your first example with the animate function immediately made me think of using it for more than just visual effects. Perhaps some sort of timing log or something along those lines.
Definitely something I’ll explore in my own code.
Cheers!
I just noticed that if you try animating an object with multiple properties, the step function is called for every increment of every property. This wasn’t quite what I was expecting. I was kind of expecting the step function to be called every time the entire object was updated (ie. one step in time).
The step function appears to be passed two arguments: the current value of the current property, and an object that holds some info about the object being animated. I’m trying to think of a neat way to detect, inside the step function, when all the properties have been updated, so I can trigger a callback on per frame rather than per step.
I just want to ask you in case you’ve already run into this and have any neat ideas?
Cheers!
I love jquery animations