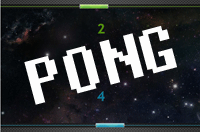
For those of you that don’t know, I’m currently studying Computer Science at the University Of Kent. As our first programming assignment we had to create a game of Pong (see screenshots here) using Java and a 2D-graphics platform called Greenfoot. It was a challenging and interesting assignment, within which I was able to discover many new things about Java and about general game development.
In my opinion, Java’s insistence on a class based approach makes it a fantastic programming language to start with, albeit being quite hefty, in terms of the sheer quantity of available classes. I also liked the fact that most of my knowledge of JavaScript could be applied to Java. There are a few syntactical discrepancies that left me confused, but all the foundation concepts were (more or less) the same.
I thought it’d be fun to port my Pong game over to JavaScript. It was painlessly quick to do actually, and it’s the first time I’ve ever built a game with JavaScript and the HTML5 canvas tag/API. You can head over to the demo to play it for yourself, and feel free to have a look at the source code. It’s not quite as sophisticated as the Java one, I didn’t have time to add cool graphics or a scoring mechanism. And yes, I know it’s the wrong way round – we were told to do it that way!
What I discovered
It’s a frame-by-frame thing
One of my very first revelations was that, to create a game, it’s important to think about everything on a frame-by-frame basis. Every new frame is a fresh slate upon which you reflect the various states represented in your program. The relationship between any two frames depends on a persisting programmable interface that “remembers” previous states.
It all becomes wonderfully simply when you think about it in this way… move the ball one cell to the left, if its position is equal to zero then it must be at the left wall, therefore we can bounce it off the wall! (okay, so it’s not actually that simple – you have to take the ball’s width into account)
It’s a massive contrast from ‘event-driven’ development, wherein you wait for the user to make a choice and then carry out something following that. In the Pong game, I’m not waiting for the user to do anything – on every new frame I simply query the necessary information to make stuff happen. For example, If the left cursor key is currently down then move this (paddle) one cell to the left! (I’m not waiting for the user to press the left cursor key, each frame is being drawn regardless.)
Everything’s an object
In my Pong game, each physical object in the game is represented as an object within the programmable layer, each one an instance of a predefined class. For example, there’d be one Paddle class from which two Paddle instances (“objects”) would be created, each representing a single physical paddle on the screen.
OOP really shines here!
Abstract, but not too much!
Abstraction really is very important, especially when there are tonnes of things that need to be happening at any time. What I actually mean here, is that it’s important to separate out functionality between your methods. This is obviously better:
if ( this.atSideEdge() ) { // ... } |
… than this:
if (curX <= halfBallWidth || curX >= worldWidth - halfBallWidth) { // ... } |
Although, this is obviously a bit too far:
if ( this.isCurrentlyWithinFivePixelsOfLeftEdge() ) { // ... } |
Game development used to be scary!
The idea of it used to be quite daunting. I feel that creating a game of Pong has opened my mind a little more to the paradigms involved in game development. It’s still a bit scary though…
Thanks for reading! Please share your thoughts with me on Twitter. Have a great day!
Nice work James – your JavaScript is always so nice to read; I’m sure your tutor will appreciate your approach to well-written code.
All the best with the course – stick at it 😉
This is pretty cool James. Good work!
Glad to hear your enjoying the work now, it sounded worrying at first from your Tweets that it maybe a bit on the “simple” side atm.
A pleasure to read your gorgeous javascript code. Very educational. Thanks!
A few years ago I made Tetris in JS and also found it handy to think in terms of frames.
By the way, I just discovered your JS syntax highlighter thingumy. Nice.