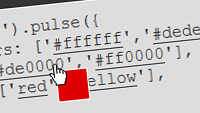
Recently, I created a brand new jQuery plugin which I wrote about on my other blog. When I release something new I like to dress it up a bit so I created a plugin page just featuring this new creation. I decided to add a couple of little enhancements to help users when viewing this page.
Tabbed Content
I needed to create a tabbed-content feature so that users would find it easier to navigate through the demos, this is how I did it: (No bloated plugins needed)
// Create new navigation menu: $('ul#demos').before('<ul id="tabs"/>'); // For each #demos list item: $('ul#demos > li').each(function(i){ // Add new list item to navigation: $('ul#tabs').append('<li><a href="#tab' + i + '">' + $('h2',this).text() + '</a></li>'); }).not(':eq(0)').css({display:'none'}); // < hide all tab contents except the first $('ul#tabs a').click(function(){ // Remove "active" class from ALL: $('#tabs > li a').removeClass('active'); // Add "active" class to the one just clicked on: $(this).addClass('active'); // Hide all tab content $('#demos > li').css({display:'none'}); // Show requested tab: (Set to display:block;) $('#demos > li:eq(' + $(this).attr('href').split('#tab')[1] + ')').css({display:'block'}); // Prevent default action: return false; }); |
Mouseover colours
I don’t think anyone knows all the HEX values of colours by heart so I made a great little feature which allows people to mouseover colours and have them appear in little floating boxes (e.g. Hover over this: #FF0000
or this: rgb(0,255,100)
) . The way it works is very simple:
// First, create a new element and append it to 'body' $('<div class="color-tip"/>').css({ position: 'absolute', zIndex: 999, border: '1px solid white', width: '30px', height: '30px', display: 'none' }).appendTo('body'); // Select all spans with a class of 'color' $('span.color').css({ cursor:'pointer', textDecoration:'underline' }).hover(function(e){ // On mouseover, show the color-tip and set background to text within the span: $('.color-tip').css({ background: $(this).text(), display: 'block' }); },function(){ // On mouseout hide the color-tip: $('.color-tip').css({display:'none'}); }).mousemove(function(e){ // When the mouse is moved on this element, move color-tip accordingly: $('.color-tip').css({ top: e.pageY+5+'px', left: e.pageX+5+'px' }); }); |
To make use of the above code simply add a class of ‘color’ to span
s with a color in:
<span class="color">#FF0000</span> <span class="color">red</span> <span class="color">rgb(255,0,0)</span> |
Note that it will only work as long as the text within each span
is an actual colour.
Thanks for reading! Please share your thoughts with me on Twitter. Have a great day!