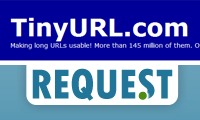
Recently I’ve been non-stop with JSONP, I love and hate it! (to find out why, see my last post!)
Anyway, David Walsh recently posted a new How-to tip on his site: "Create a TinyURL with PHP" – His tutorial taps into the TinyURL API to retrieve the shortened URL which you can then display on a page.
For those that don’t want to get their hands dirty with PHP there is a very simple way to do it with just JavaScript, Ajax is not needed!
Here we go:
Using [one of] the TinyURL API[s]:
function getTinyURL(longURL, success) { // Create unique name for callback function: var ud = 'json'+(Math.random()*100).toString().replace(/./g,''), // Define API URL: API = 'http://json-tinyurl.appspot.com/?url='; // Define a new global function: // (which will run the passed 'success' function: window[ud]= function(o){ success&&success(o.tinyurl); }; // Append new SCRIPT element to BODY with SRC of API: document.getElementsByTagName('body')[0].appendChild((function(){ var s = document.createElement('script'); s.type = 'text/javascript'; s.src = API + encodeURIComponent(longURL) + '&callback=' + ud; return s; })()); } getTinyURL('https://j11y.io/javascript/cross-domain-requests-with-jsonp-safe/', function(tinyurl){ // Do something with tinyurl: alert(tinyurl) }); |
And with jQuery:
function getTinyURL(longURL, success) { var API = 'http://json-tinyurl.appspot.com/?url=', URL = API + encodeURIComponent(longURL) + '&callback=?'; $.getJSON(URL, function(data){ success && success(data.tinyurl); }); } getTinyURL('https://j11y.io/javascript/cross-domain-requests-with-jsonp-safe/', function(tinyurl){ // Do something with tinyurl: alert(tinyurl) }); |
Using the Reque.st API:
I’ve made it possible to get JSONP output through the reque.st API too, so we can do something similar to get a shorter ‘reque.st’ URL:
function requestShortURL(longURL, success) { var ud = 'json'+(Math.random()*100).toString().replace(/./g,''), API = 'http://reque.st/create.api.php?json&url='; window[ud]= function(o){ success&&success(o.url); }; document.getElementsByTagName('body')[0].appendChild((function(){ var s = document.createElement('script'); s.type = 'text/javascript'; s.src = API + encodeURIComponent(longURL) + '&callback=' + ud; return s; })()); } requestShortURL('https://j11y.io/javascript/cross-domain-requests-with-jsonp-safe/', function(shortened){ // Do something with shortened URL: alert(shortened) }); |
And with jQuery:
function requestShortURL(longURL, success) { var API = 'http://reque.st/create.api.php?json&url=', URL = API + encodeURIComponent(longURL) + '&callback=?'; $.getJSON(URL, function(data){ success && success(data.url); }); } requestShortURL('https://j11y.io/javascript/cross-domain-requests-with-jsonp-safe/', function(shortened){ // Do something with shortened URL: alert(shortened) }); |
So, as you can see, it’s very simple! If you have no idea what just happened then I suggest reading up on JSONP, here (http://bob.pythonmac.org/archives/2005/12/05/remote-json-jsonp/), here (http://www.west-wind.com/Weblog/posts/107136.aspx) and here (http://remysharp.com/2007/10/08/what-is-jsonp/).
I know I said the last post would be the last one of 2008 but I suddenly had a JSONP moment which just couldn’t go undocumented! 🙂
MERRY CHRISTMAS!
Thanks for reading! Please share your thoughts with me on Twitter. Have a great day!
Hi, do you mind if I use this JSONP API for creating short urls?
Sorry for the late reply Alex. Yep that’s absolutely fine! 🙂
Hi, James!
I used bits of your code to create a twitter+tinyurl bookmarklet for my own convenience 🙂 I hope you don’t mind! Check it out here: http://gricode.tumblr.com/post/75503433/twitter-tinyurl-bookmarklet
cheers,
Grigory.